In this article we are going to write the our own implementation for Solr repository and add some custom methods to it, However I am going to cover every little details but you should have little bit idea of Apache Solr, Spring Boot Repositories to understand this article.
Apache Solr is basically an open source web application built on top of Apache lucene search API but Solr provides lots of extra functionalities than lucene. You don't need to be a Java programmer to perform search on Solr it provides a cool user interface with help of its REST-like APIs, We can do approx everything on this UI without having any knowledge of programming with little bit configuration. Rather than this Solr also provides advanced and optimised full text search, integration with Apache UIMA and Apache Tika and lots more you can see them official website of solr.
With initiations convention over configuration Spring introduced a new framework named Spring Boot, Spring Boot has a lots of advance thing and one of them is the concept of repositories. Spring boot Repositories have some methods which are commonly used while writing any enterprise apps.
Here we are going to use Spring boot Solr repository and provide some custom implementation for that repository.
First of all we need to setup a rough maven project structure, we can generate and download it from Spring Initializer or we can create it.
Now let's move our demo application, here I am going to create complete project from scratch in below simple steps.
Step 1. Create A maven project with Java 8 archtype
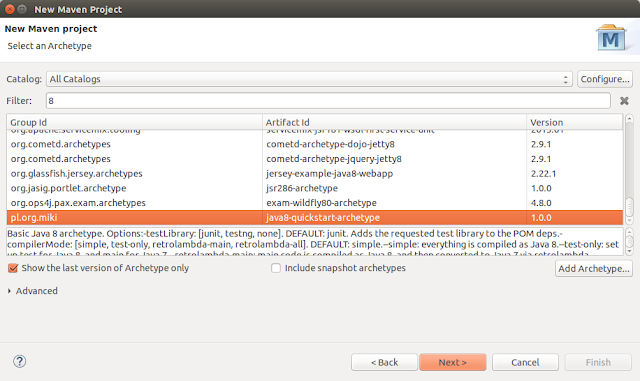
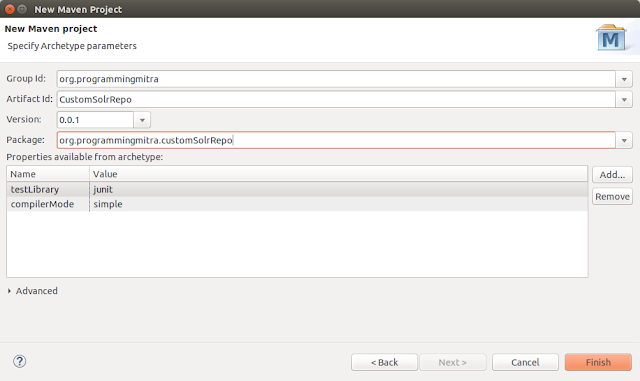
Step 2. Download, install and configure Apache Solr Http server, and create a core. I have created a core by name basic_core by using basic_configs.
Step 3. Add spring-boot-starter and spring-data-solr dependencies to your pom.xml file.
Step 4. Create below configuration classes and add solr.server.url=http://localhost:8983/solr/basic_core to application.properties file under resources folder.
CustomSolrRepoApp.java
Marking our class with @SpringBootApplication is same as marking the class with @Documented, @Inherited, @Configuration, @EnableAutoConfiguration, @ComponentScan annotations
It is not general practice to write any code in this class rather than
SpringApplication.run(CustomSolrRepoApp.class, args);
But in this class I am getting the repository bean from application context and then creating dummy data with help of that repository instance and then calling findall() method of SolrRepository class and also our custom getLastNames() method which will give us unique list of lastnames present in our dummy data.
SolrConfig.java
Here we are creating beans for SolrServer and SolrTemplate, Here we are using HttpSolrServer to communicate with Apache solr, however we can also use EmbeddedSolrServerFactoryBean for same purpose. EmbeddedSolrServerFactoryBean is only good for dev server not production server.
Step 5. Create Employee model class
Employee.java
Mark id field with @Id and @Field annotation and other fields with @Field annotation
Step 6. Create repositories, custom repository and repository implementations
CustomSolrRepository.java
Define all your custom methods in this interface
EmployeeRepository.java
This class extends CustomSolrRepository and SolrCrudRepository interface here, This interface will be treated as Solr repository reference
EmployeeRepositoryImpl.java
This should be named as EmployeeRepository + impl according spring boot repository name convention. This class should also marked as @Repository annotation and it should also provide implementations for CustomSolrRepository's methods
To see the output we need to run CustomSolrRepoApp as java application as we can see in below screenshot first we will have the complete list of employees and then we will have list of unique last names.
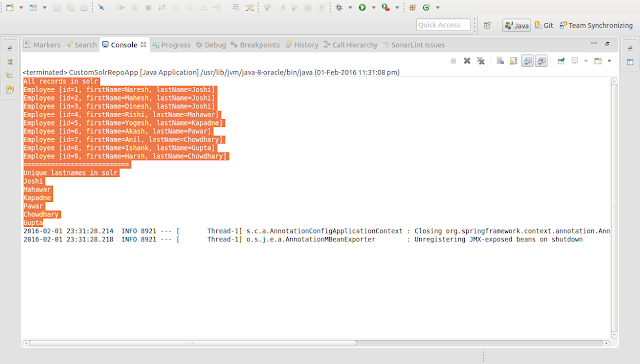
At the end I want to say thanks for reading, Please feel free to contact me if you found anything wrong or phase any other problem, or if you just want to talk.
Apache Solr is basically an open source web application built on top of Apache lucene search API but Solr provides lots of extra functionalities than lucene. You don't need to be a Java programmer to perform search on Solr it provides a cool user interface with help of its REST-like APIs, We can do approx everything on this UI without having any knowledge of programming with little bit configuration. Rather than this Solr also provides advanced and optimised full text search, integration with Apache UIMA and Apache Tika and lots more you can see them official website of solr.
With initiations convention over configuration Spring introduced a new framework named Spring Boot, Spring Boot has a lots of advance thing and one of them is the concept of repositories. Spring boot Repositories have some methods which are commonly used while writing any enterprise apps.
Here we are going to use Spring boot Solr repository and provide some custom implementation for that repository.
First of all we need to setup a rough maven project structure, we can generate and download it from Spring Initializer or we can create it.
Now let's move our demo application, here I am going to create complete project from scratch in below simple steps.
Step 1. Create A maven project with Java 8 archtype
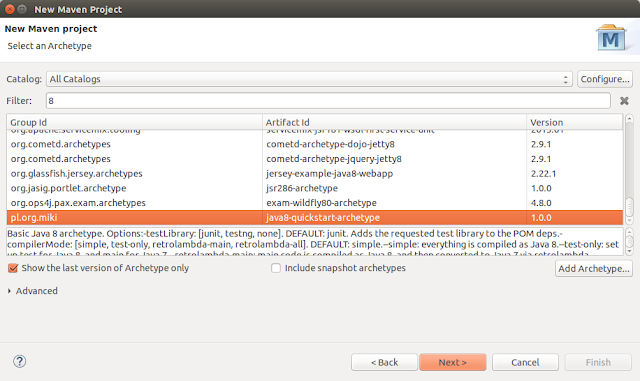
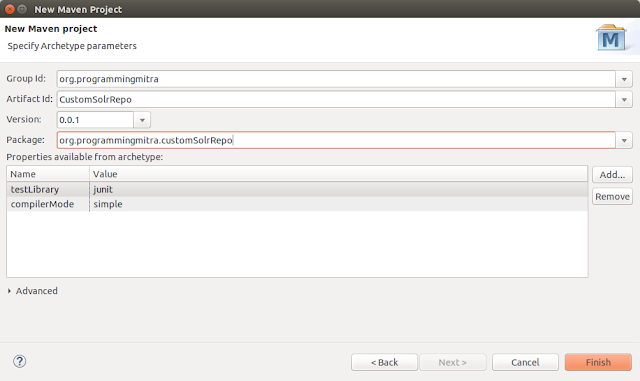
Step 2. Download, install and configure Apache Solr Http server, and create a core. I have created a core by name basic_core by using basic_configs.
Step 3. Add spring-boot-starter and spring-data-solr dependencies to your pom.xml file.
Step 4. Create below configuration classes and add solr.server.url=http://localhost:8983/solr/basic_core to application.properties file under resources folder.
CustomSolrRepoApp.java
Marking our class with @SpringBootApplication is same as marking the class with @Documented, @Inherited, @Configuration, @EnableAutoConfiguration, @ComponentScan annotations
It is not general practice to write any code in this class rather than
SpringApplication.run(CustomSolrRepoApp.class, args);
But in this class I am getting the repository bean from application context and then creating dummy data with help of that repository instance and then calling findall() method of SolrRepository class and also our custom getLastNames() method which will give us unique list of lastnames present in our dummy data.
SolrConfig.java
Here we are creating beans for SolrServer and SolrTemplate, Here we are using HttpSolrServer to communicate with Apache solr, however we can also use EmbeddedSolrServerFactoryBean for same purpose. EmbeddedSolrServerFactoryBean is only good for dev server not production server.
Step 5. Create Employee model class
Employee.java
Mark id field with @Id and @Field annotation and other fields with @Field annotation
Step 6. Create repositories, custom repository and repository implementations
CustomSolrRepository.java
Define all your custom methods in this interface
EmployeeRepository.java
This class extends CustomSolrRepository and SolrCrudRepository interface here, This interface will be treated as Solr repository reference
EmployeeRepositoryImpl.java
This should be named as EmployeeRepository + impl according spring boot repository name convention. This class should also marked as @Repository annotation and it should also provide implementations for CustomSolrRepository's methods
To see the output we need to run CustomSolrRepoApp as java application as we can see in below screenshot first we will have the complete list of employees and then we will have list of unique last names.
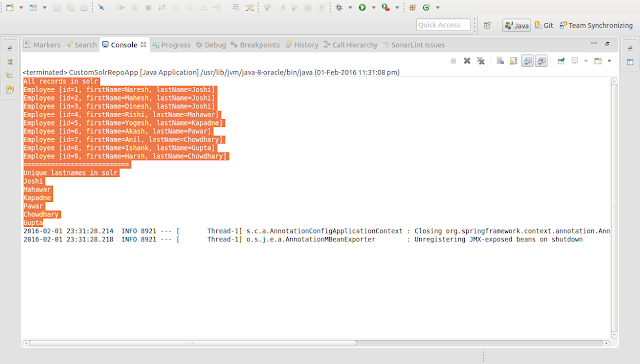
At the end I want to say thanks for reading, Please feel free to contact me if you found anything wrong or phase any other problem, or if you just want to talk.