Git is a most widely used and powerful version control system for tracking changes in computer files and coordinating work on those files among multiple people. It is primarily used for source code management in software development, but it can be used to keep track of changes in any set of files.
Git was developed by Linus Torvalds in 2005 as a distributed open-source software version control software and of course, it is free to use. As a distributed revision control system it is aimed at speed, data integrity, and support for distributed, non-linear workflows.
While other version control systems e.g. CVS, SVN keeps most of their data like commit logs on a central server, every git repository on every computer is a full-fledged repository with a complete history and full version tracking abilities, independent of network access or a central server.
However, almost all IDEs support git out of the box and we do not require submitting the git commands manually but it is always good to understand these commands. Below is a list of some git commands to work efficiently with git.
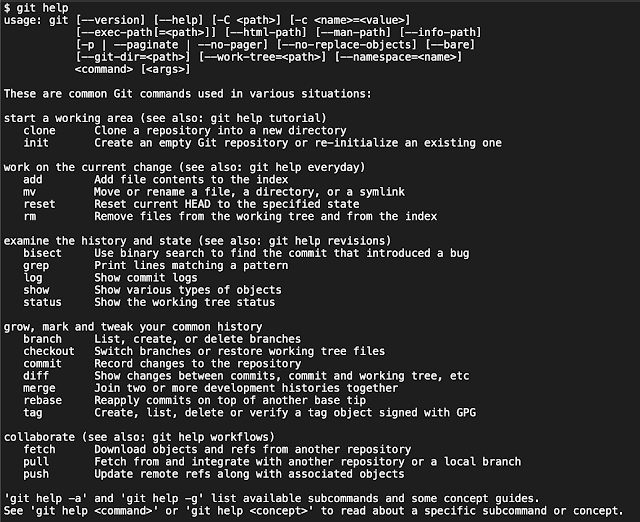
Command
And command
We can use
I keep these commands as notes for my future reference and you can find more on this Github Repository and please feel free to provide your valuable feedback.
Git was developed by Linus Torvalds in 2005 as a distributed open-source software version control software and of course, it is free to use. As a distributed revision control system it is aimed at speed, data integrity, and support for distributed, non-linear workflows.
While other version control systems e.g. CVS, SVN keeps most of their data like commit logs on a central server, every git repository on every computer is a full-fledged repository with a complete history and full version tracking abilities, independent of network access or a central server.
However, almost all IDEs support git out of the box and we do not require submitting the git commands manually but it is always good to understand these commands. Below is a list of some git commands to work efficiently with git.
Git Help
The most useful command in git isgit help
which provides us with all the help we require. If we type git help
in the terminal, we will get: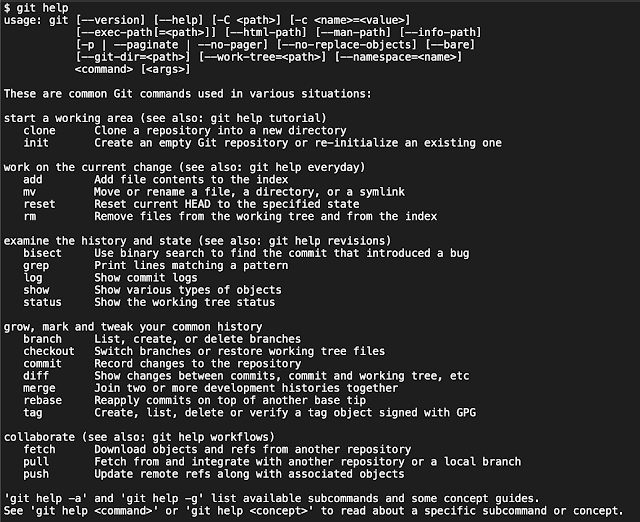
Command
git help -a
will give us a complete list of git commands:Available git commands in '/usr/local/git/libexec/git-core'
add gc receive-pack
add--interactive get-tar-commit-id reflog
am grep remote
annotate gui remote-ext
apply gui--askpass remote-fd
archimport gui--askyesno remote-ftp
archive gui.tcl remote-ftps
askpass hash-object remote-http
bisect help remote-https
bisect--helper http-backend repack
blame http-fetch replace
branch http-push request-pull
bundle imap-send rerere
cat-file index-pack reset
check-attr init rev-list
check-ignore init-db rev-parse
check-mailmap instaweb revert
check-ref-format interpret-trailers rm
checkout log send-email
checkout-index ls-files send-pack
cherry ls-remote sh-i18n--envsubst
cherry-pick ls-tree shortlog
citool mailinfo show
clean mailsplit show-branch
clone merge show-index
column merge-base show-ref
commit merge-file stage
commit-tree merge-index stash
config merge-octopus status
count-objects merge-one-file stripspace
credential merge-ours submodule
credential-manager merge-recursive submodule--helper
credential-store merge-resolve subtree
credential-wincred merge-subtree svn
cvsexportcommit merge-tree symbolic-ref
cvsimport mergetool tag
daemon mktag unpack-file
describe mktree unpack-objects
diff mv update
diff-files name-rev update-git-for-windows
diff-index notes update-index
diff-tree p4 update-ref
difftool pack-objects update-server-info
difftool--helper pack-redundant upload-archive
fast-export pack-refs upload-pack
fast-import patch-id var
fetch prune verify-commit
fetch-pack prune-packed verify-pack
filter-branch pull verify-tag
fmt-merge-msg push web--browse
for-each-ref quiltimport whatchanged
format-patch read-tree worktree
fsck rebase write-tree
fsck-objects rebase--helper
And command
git help -g
will give us a list of git concepts which git think is good for us:The common Git guides are:
attributes Defining attributes per path
everyday Everyday Git With 20 Commands Or So
glossary A Git glossary
ignore Specifies intentionally untracked files to ignore
modules Defining submodule properties
revisions Specifying revisions and ranges for Git
tutorial A tutorial introduction to Git (for version 1.5.1 or newer)
workflows An overview of recommended workflows with Git
We can use
git help <command>
or git help <concept>
command to know more about a specific command or concept.Git Configuration
Description | Git Command |
---|---|
List all configurations applied to the current repository in order (picked from bottom to top). | git config --list Or git config --global --list |
Configure the author name to be used with your commits by replacing old entries. | git config --global --replace-all user.name "Sam Smith" |
Configure the author email address to be used with your commits | git config --global user.email [email protected] |
Will remove user credential details from the repository | git config --local credential.helper "" |
List all currently configured remote repository URLs | git remote -v |
If you haven't connected your local repository to a remote server, To add a remote server to a local repository | git remote add origin <repo_url> |
Git Commit and Push
Description | Git Command |
---|---|
Create a file name README.md with Readme content content | echo "Readme content" >> README.md |
List the files you've changed and those you still need to add or commit | git status |
Add all or one file to the staging area | git add . OR git add folder/file_name |
Remove all or one file from the staging area | git reset HEAD OR git reset HEAD folder/file_name |
Commit changes to head with a message | git commit -m 'message' |
Commit any files you've added with git add , and also commit any files you've changed since then | git commit -a |
Send all commits from local repository to remote repository | git push |
Do a git push and sets the default remote branch for the current local branch. So any future git pull command will attempt to bring in commits from the <remote-branch> into the current local branch | git push -u <remote-branch> |
Send changes to the master branch of your remote repository | git push origin master |
Push a specific branch to your remote repository | git push origin <branch_name> |
Push all branches to your remote repository | git push --all origin |
Git Checkout And Pull
Description | Git Command |
---|---|
To create a new local repository | git init |
Clone a repository into a new directory | git clone repo_url |
Clone a repository into a new directory and point to mentioned branch_name | git clone --branch branch_name repo_url |
To create a working copy of a local repository | git clone /path/to/repository |
Download objects and refs from a remote repository for the master branch | git fetch origin master |
To merge a different branch into your active branch | git merge <branch_name> |
Fetch and merge changes on the remote server to your working directory: | git pull |
View all the merge conflicts, View the conflicts against the base file, Preview changes, before merging | git diff , git diff --base <filename> , git diff <sourcebranch> <targetbranch> |
Git Branch
Description | Git Command |
---|---|
List all the branches in your repository, and also tell you what branch you're currently in | git branch |
Switch from one branch to another | git checkout branch_name |
Create a new branch and switch to it | git checkout -b branch_name |
Create a new branch from the master branch and switch to it | git checkout -b branch_name master |
Delete the feature branch from the local repository | git branch -d <branch_name> |
Delete a branch on your remote repository | git push origin :<branch_name> |
Git Cleaning
Description | Git Command |
---|---|
Fetch the latest history (objects & refs) from the remote server for the master branch | git fetch origin master |
Clean the repository to the last push | git clean -x -d -f |
Reset local repository and point your local master branch to latest history fetched from the remote server | git reset --hard origin/master |
To bring all changes from a remote repository to the local repository | git pull origin master |
Other Git commands
Description | Git Command |
---|---|
You can use tagging to mark a significant changeset, such as a release | git tag 1.0.0 <commitID> |
Commit Id is the leading characters of the changeset ID, up to 10, but must be unique. Get the ID using | git log |
Push all tags to the remote repository | git push --tags origin |
If you mess up, you can replace the changes in your working tree with the last content in head: Changes already added to the index, as well as new files, will be kept | git checkout -- <filename> |
Search the working directory for foo() | git grep "foo()" |
I keep these commands as notes for my future reference and you can find more on this Github Repository and please feel free to provide your valuable feedback.