In this article, we will see How Does JVM Handle Method Overloading And Overriding Internally, how JVM identifies which method should get called.
Let’s take the example of a parent class
Mammal
and a child Human
classes from our previous blog to understand it more clearly.public class OverridingInternalExample {
private static class Mammal {
public void speak() { System.out.println("ohlllalalalalalaoaoaoa"); }
}
private static class Human extends Mammal {
@Override
public void speak() { System.out.println("Hello"); }
// Valid overload of speak
public void speak(String language) {
if (language.equals("Hindi")) System.out.println("Namaste");
else System.out.println("Hello");
}
@Override
public String toString() { return "Human Class"; }
}
// Code below contains the output and and bytecode of the method calls
public static void main(String[] args) {
Mammal anyMammal = new Mammal();
anyMammal.speak(); // Output - ohlllalalalalalaoaoaoa
// 10: invokevirtual #4 // Method org/programming/mitra/exercises/OverridingInternalExample$Mammal.speak:()V
Mammal humanMammal = new Human();
humanMammal.speak(); // Output - Hello
// 23: invokevirtual #4 // Method org/programming/mitra/exercises/OverridingInternalExample$Mammal.speak:()V
Human human = new Human();
human.speak(); // Output - Hello
// 36: invokevirtual #7 // Method org/programming/mitra/exercises/OverridingInternalExample$Human.speak:()V
human.speak("Hindi"); // Output - Namaste
// 42: invokevirtual #9 // Method org/programming/mitra/exercises/OverridingInternalExample$Human.speak:(Ljava/lang/String;)V
}
}
We can answer this answer in two ways, Logical way and Physical way, let's take a look at the logical way.
Logical Way
Logically we can say, during compilation phase calling method is considered from the reference type. But at execution time method will be called from the object which the reference is holding.For Example on
humanMammal.speak();
line compiler will say
Mammal.speak()
is getting called because
humanMammal
is of type
Mammal
. But during execution, JVM knows that
humanMammal
is holding a
Human's
object so
Human.speak()
will get called.Well, it is pretty simple until we keep it at the conceptual level only. Once we get the doubt that how JVM is handling all this internally? or how JVM is calculating which method it should call.
Also, we know that overloaded methods are not called polymorphic and get resolved at compile time and this is why sometimes method overloading is also known as compile time polymorphism or early/static binding.
But overridden methods get resolved at runtime time because the compiler does not know that, the object which we are assigning to our reference have overridden the method or not.
Physical Way
In this section, we will try to find out physical proof of all aforementioned statements and to find them we will read the bytecode of our program which we can do by executing
javap -verbose OverridingInternalExample
. By using
-verbose
option we will get the descriptive bytecode same as our Java program.Above command shows the bytecode in two sections
1. Constant Pool: holds almost everything which is necessary for our program’s execution e.g. method references (
#Methodref
), Class objects (
#Class
), string literals (
#String
), please click one image to zoom.
2. Program’s Bytecode: executable bytecode instructions, please click one image to zoom.

Why Method overloading is called static binding
In the above mention code
humanMammal.speak()
compiler will say
speak()
is getting called from
Mammal
but at execution time it will be called from the object which
humanMammal
is holding, which is the object of the Human
class.And by looking at the above code and images we can see that the bytecodes of
humanMammal.speak()
,
human.speak()
and
human.speak("Hindi")
are totally different because the compiler is able to differentiate between them based on the class reference.So in the case of method overloading compiler is able to identify the bytecode instructions and method’s address at compile time and that is why it is also known as static binding or compile time polymorphism.
Why Method overriding is called dynamic binding
Bytecode for
anyMammal.speak()
and
humanMammal.speak()
are same (
invokevirtual #4 // Method org/programming/mitra/exercises/OverridingInternalExample$Mammal.speak:()V
) because according to compiler both methods are called on
Mammal
reference.So now the question comes if both method calls have same bytecode then how does JVM know which method to call?
Well, the answer is hidden in the bytecode itself and it is
invokevirtual
instruction, according to JVM specificationinvokevirtual invokes an instance method of an object, dispatching on the (virtual) type of the object. This is the normal method dispatch in the Java programming language.
JVM uses the
invokevirtual
instruction to invoke Java equivalent of the C++ virtual methods. In C++ if we want to override one method in another class we need to declare it as
virtual
, But in Java, all methods are virtual by default (except final and static methods) because we can override every method in the child class.Operation
invokevirtual
accepts a pointer to method reference call (
#4
an index into the constant pool)invokevirtual #4 // Method org/programming/mitra/exercises/OverridingInternalExample$Mammal.speak:()V
And that method reference #4 again refers to a method name and Class reference
#4 = Methodref #2.#27 // org/programming/mitra/exercises/OverridingInternalExample$Mammal.speak:()V
#2 = Class #25 // org/programming/mitra/exercises/OverridingInternalExample$Mammal
#25 = Utf8 org/programming/mitra/exercises/OverridingInternalExample$Mammal
#27 = NameAndType #35:#17 // speak:()V
#35 = Utf8 speak
#17 = Utf8
()V
All these references combinedly used to get a reference to a method and class in which the method is to be found. This is also mentioned in JVM Specification
The Java virtual machine does not mandate any particular internal structure for objects 4.
And the bookmark 4 states
In some of Oracle’s implementations of the Java virtual machine, a reference to a class instance is a pointer to a handle that is itself a pair of pointers: one to a table containing the methods of the object and a pointer to the Class object that represents the type of the object, and the other to the memory allocated from the heap for the object data.
It means every reference variable holds two hidden pointers
- A pointer to a table which again holds methods of the object and a pointer to the Class object. e.g. [speak(), speak(String) Class object]
- A pointer to the memory allocated on the heap for that object’s data e.g. values of instance variables.
invokevirtual
internally do this? Well, no one can answer this because it depends on JVM implementation and it varies from JVM to JVM.And from the above statements, we can conclude that an object reference indirectly holds a reference/pointer to a table which holds all the method references of that object. Java has borrowed this concept from C++ and this table is known by various names which such as virtual method table ( VMT ), virtual function table (vftable), virtual table (vtable), dispatch table.
We can not sure of how
vtable
is implemented in Java because it is JVM dependent. But we can expect that it will be following the same strategy as C++ where
vtable
is an array like structure which holds method names and their references on array indices. And whenever JVM tries to execute a virtual method it always asks the
vtable
for it address.There is only one
vtable
per class, which means it is unique and same for all objects of a class similar to Class
object. I have discussed more on
Class
object in my articles Why an outer Java class can’t be static and Why Java is Purely Object-Oriented Language Or Why Not.So there is only one
vtable
for
Object
class which contains all 11 methods (if we don't count registerNatives) and references to their respective method bodies.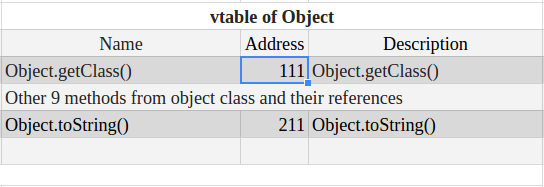
When JVM loads the
Mammal
class into memory it creates a
Class
object for it and creates a
vtable
which contains all the methods from the vtable of Object class with the same references (Because
Mammal
is not overriding any method from Object) and adds a new entry for
speak
method.
Now here comes the turn of
Human
class and now JVM will copy all entries from the
vtable
of
Mammal
class to the
vtable
of
Human
and adds a new entry for the overloaded version of
speak(String)
.JVM knows that
Human
class has overridden two methods one is
toString()
from
Object
and second is
speck()
from
Mammal
. Now instead of creating new entries for these methods with updated references. JVM will modify the references to the already present methods on the same index where they were present earlier and will keep the same method names.
The
invokevirtual
causes the JVM to treat the value at method reference
#4
, not as an address but as the name of a method to look up in the
vtable
for the current object.I hope now it would have become a little bit clear that how the JVM mixes
constant pool
entries and
vtable
to conclude which method it is going to call.You can find the complete code on this Github Repository and please feel free to provide your valuable feedback.
Good post.💪
ReplyDeleteThanks Chamini
DeleteWell Explained Naresh
ReplyDeleteThanks Prashant
DeleteGreat job Naresh!!!! ... I am not so good at Java, but after reading your blog, it easily comes to understand. You easily explain the complexities of all topics.
ReplyDeleteThanks Amritanjali
DeleteVery nice post.
ReplyDeleteThank you for sharing knowledge.
Thanks Pradip!
Deletevery good thank you
ReplyDeleteThanks Mohammed!
DeleteReally good article, but I'm wondering how invoke of super.speak() in class Human is handled. If in Human vtable overriden methods overrides orginal Mammal methods references, then we lose information about original reference to Mammal.speak() method. Hence, in my opinion, we cannot invoke super.speak() from Human class.
ReplyDelete
DeleteThanks for your feedback, super keyword always represents the instance of parent class (Mammal) and it does not care about what child (Human) is overriding and what not. So any method call on super instance will do a lookup on Parent class's vtable not child's.
The super.f() use JVM instruction invokespecial, it's different from invokevirtual. See below example:
Deleteclass Parent {
void f() {}
}
class Child extends Parent {
@Override
void f() {
super.f();
}
}
javap -c Child.class you will got:
class Child extends Parent {
void f();
Code:
0: aload_0
1: invokespecial #2 // Method Parent.f:()V
4: return
}
Yes Indeed1
DeleteHi Naresh,
ReplyDeleteWhen exactly an Object reference will be created? Is it during the compile time, where bytecode gets generated? Or when the JVM processes the bytecode? Kindly clarify.
Ideally it should happen at runtime when objects get created then only there references get created.
Delete/
ReplyDeleteThanks Naresh, this was really helpful in gaining a deeper understanding of how Java works!
ReplyDeleteThanks!
DeleteThanks for clearing it so well.
ReplyDeleteMalatya
ReplyDeleteKırıkkale
Aksaray
Bitlis
Manisa
GE8L
bitlis
ReplyDeletesakarya
van
tunceli
ankara
6Yİ0K
Bolu Lojistik
ReplyDeleteMardin Lojistik
Kocaeli Lojistik
Diyarbakır Lojistik
İstanbul Lojistik
VR1A
F6CF9
ReplyDeleteBitcoin Nasıl Alınır
Tokat Parça Eşya Taşıma
Aydın Şehirler Arası Nakliyat
Sivas Lojistik
Azero Coin Hangi Borsada
Yenimahalle Boya Ustası
Uşak Lojistik
Çerkezköy Çilingir
Nexa Coin Hangi Borsada
D4A6E
ReplyDeletekomisyon indirimi %20
60268
ReplyDeletemalatya muhabbet sohbet
bedava görüntülü sohbet
mersin telefonda görüntülü sohbet
balıkesir parasız sohbet siteleri
rastgele sohbet siteleri
mardin görüntülü sohbet ücretsiz
ücretsiz sohbet sitesi
adana ücretsiz sohbet sitesi
sakarya goruntulu sohbet
3BAC1
ReplyDeletemuğla telefonda canlı sohbet
antalya canlı görüntülü sohbet siteleri
izmir sesli mobil sohbet
kadınlarla sohbet
Aksaray Sesli Sohbet Odası
Bartın Parasız Sohbet Siteleri
telefonda kızlarla sohbet
Gümüşhane Ücretsiz Sohbet Sitesi
bitlis görüntülü sohbet sitesi
ACA16
ReplyDeletenevşehir mobil sohbet siteleri
görüntülü sohbet kadınlarla
Osmaniye Canlı Sohbet Siteleri
Kastamonu Ücretsiz Sohbet Uygulaması
aydın rastgele sohbet odaları
ücretsiz sohbet sitesi
Afyon Random Görüntülü Sohbet
rize rastgele canlı sohbet
muş sesli sohbet uygulamaları
F8B44
ReplyDeleteantalya görüntülü sohbet ücretsiz
kilis en iyi ücretsiz sohbet siteleri
Mardin Parasız Görüntülü Sohbet
Istanbul Kadınlarla Ücretsiz Sohbet
mardin en iyi görüntülü sohbet uygulaması
aksaray canlı görüntülü sohbet uygulamaları
sivas canlı sohbet
Düzce Ücretsiz Sohbet
amasya en iyi rastgele görüntülü sohbet
5A1C9
ReplyDeletemuğla canlı sohbet bedava
sesli mobil sohbet
Giresun Sesli Mobil Sohbet
yabancı görüntülü sohbet siteleri
Ordu Canlı Görüntülü Sohbet Odaları
bedava görüntülü sohbet sitesi
manisa yabancı sohbet
balıkesir sesli mobil sohbet
Adıyaman Mobil Sohbet Odaları
828CA
ReplyDeleteBinance Referans Kodu
Linkedin Takipçi Satın Al
Binance Referans Kodu
Soundcloud Takipçi Satın Al
Bee Coin Hangi Borsada
Bitcoin Nasıl Oynanır
Twitch İzlenme Hilesi
Btcst Coin Hangi Borsada
Okex Borsası Güvenilir mi
0F324
ReplyDeletecopy trade nedir
bitcoin nasıl oynanır
bitrue
bitexen
vindax
bitcoin nasıl kazanılır
canlı sohbet odaları
binance
bitexen
68429
ReplyDeletebitcoin seans saatleri
https://toptansatinal.com/
kucoin
papaya
bybit
rastgele canlı sohbet
kripto para nasıl alınır
bitcoin nasıl üretilir
en iyi kripto para uygulaması
9AC54
ReplyDeletekripto ne demek
kucoin
binance
canlı sohbet odaları
4g mobil proxy
referans kimliği
filtre kağıdı
btcturk
mexc
83CEC
ReplyDeletekripto para haram mı
kraken
ilk kripto borsası
huobi
vindax
canlı sohbet odaları
bitexen
bingx
canlı sohbet
4F8DD
ReplyDeletebtcturk
mexc
bitget
kraken
canli sohbet
bitcoin nasıl kazanılır
May 2024 Calendar
bitexen
en güvenilir kripto borsası
9825B47173
ReplyDeletetelegram show
görüntülü show
cialis
görüntülü şov
maxman
skype show
telegram görüntülü şov
lady era
viagra
1F60AF2FB7
ReplyDeleteviagra
novagra
cam şov
ücretli şov
kamagra
degra
delay
skype show
ereksiyon hapı
F697D4BC05
ReplyDeletewhatsapp ücretli show
bufalo çikolata
görüntülü şov whatsapp numarası
canli cam show
geciktirici jel
whatsapp görüntülü show güvenilir
telegram görüntülü şov
kamagra hap
bufalo içecek
8D9CEA6EDE
ReplyDeleteinstagram ucuz takipçi
417301ED05
ReplyDeletetiktok takipçi atma
F0AD946382
ReplyDeletetakipci satışı
E27BD780E4
ReplyDeleteYeni Telegram Madencilik Botları
En İyi Telegram Airdrop Botları
Telegram Para Kazanma
En İyi Telegram Madencilik Botları
Telegram Airdrop Botları
8329F8733F
ReplyDeleteTelegram Güvenilir Coin Botları
Yeni Telegram Coin Kasma Botları
Ton Coin Kazandıran Oyunlar
Yeni Telegram Farm Botları
Telegram Güvenilir Coin Kasma Botları